Here’s an Arduino-compatible GI-SP0256-AL2 speech synthesizer module that I’m finishing up. It’s a great 1980’s-era allophone speech synthesizer chip that was used in Intellivision expansion modules and sold at RadioShack stores for years for about $12. You can still find them from time to time on ebay, and they produce a fantastic synthesized speech sound. The chip is sometimes called the “SPO256-AL2” (with the letter “O” as opposed to the numeral “0”) due to a typo in the original documentation from RadioShack.
First, here’s a video of the speech synthesizer in action:
The chip works by sending it a series of allophones (59 to choose from) that make up all the sounds of the English language. It’s a real rats nest when wired up on a breadboard, so I thought I’d throw it together on a little 5cm circuit board. (Let me know by commenting below if you’re interested in one.)
The board leaves open (and accessible) four analog pins and four digital pins (two with PWM), plus two additional analog pins in you use the serial clock and data lines that are set up for I2C communication by default. The eight non-I2C pins are paired with ground pins, and three are set up by default to configure the device address for I2C communication. It requires regulated 5V via a standard 6-pin FTDI connection (you don’t need to use all pins unless you’re programming it), and there’s an output jumper at the bottom that can be amplified to power a speaker.
Here’s the design. It’s about 2 inches square. I’ll post the code when I get it finished up. Let me know if you’re interested!
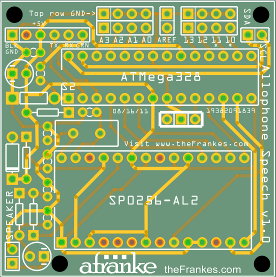
Some Code
Here’s some of the code I used for my test. I’ve updated the code on Februay 18, 2012 to include the required loop() method (which I’d accidentally left out) and to rename the “SS” constant so it doesn’t conflict with the Slave Select constant in case that’s defined.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 | // Voice Pins -- The SP0256 address pins are all on the same port here. // This isn't necessary but it does make it a lot easier to pick an // allophone in code using PORTC in this case. #define PIN_A1 A0 #define PIN_A2 A1 #define PIN_A3 A2 #define PIN_A4 A3 #define PIN_A5 A4 #define PIN_A6 A5 #define PIN_ALD 2 #define PIN_LRQ 12 // some words to say byte purple[] = {PP, ER1, PP, LL }; byte monkey[] = {MM, AX, NN1, KK1, IY }; byte garden[] = {GG1, AR, PA3, DD2, IH, NN1 }; byte moment[] = {MM, OW, MM, EH, NN1, TT2 }; void setup() { // Set pin modes pinMode( PIN_ALD, OUTPUT ); pinMode( PIN_LRQ, INPUT ); DDRC = B00111111; // Sets Analog pins 0-5 to output digitalWrite(PIN_ALD, HIGH); speak( purple, (byte)( sizeof (purple) / sizeof (byte)) ); speak( monkey, (byte)( sizeof (monkey) / sizeof (byte)) ); speak( garden, (byte)( sizeof (garden) / sizeof (byte)) ); speak( moment, (byte)( sizeof (moment) / sizeof (byte)) ); } void loop() { } void speak( byte* allophones, byte count ) { for ( byte b = 0; b < count; b++ ) { speak( allophones[b] ); } speak( PA4 ); // short pause after each word } void speak( byte allophone ) { while ( digitalRead(PIN_LRQ) == HIGH ) ; // Wait for LRQ to go low PORTC = allophone; // select the allophone // Tell it to speak by toggling ALD digitalWrite(PIN_ALD, LOW); digitalWrite(PIN_ALD, HIGH); } |
Allophones
Here are the allophone definitions:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | #define PA1 0x00 #define PA2 0x01 #define PA3 0x02 #define PA4 0x03 #define PA5 0x04 #define OY 0x05 #define AY 0x06 #define EH 0x07 #define KK3 0x08 #define PP 0x09 #define JH 0x0A #define NN1 0x0B #define IH 0x0C #define TT2 0x0D #define RR1 0x0E #define AX 0x0F #define MM 0x10 #define TT1 0x11 #define DH1 0x12 #define IY 0x13 #define EY 0x14 #define DD1 0x15 #define UW1 0x16 #define AO 0x17 #define AA 0x18 #define YY2 0x19 #define AE 0x1A #define HH1 0x1B #define BB1 0x1C #define TH 0x1D #define UH 0x1E #define UW2 0x1F #define AW 0x20 #define DD2 0x21 #define GG3 0x22 #define VV 0x23 #define GG1 0x24 #define SH 0x25 #define ZH 0x26 #define RR2 0x27 #define FF 0x28 #define KK2 0x29 #define KK1 0x2A #define ZZ 0x2B #define NG 0x2C #define LL 0x2D #define WW 0x2E #define XR 0x2F #define WH 0x30 #define YY1 0x31 #define CH 0x32 #define ER1 0x33 #define ER2 0x34 #define OW 0x35 #define DH2 0x36 #define SSS 0x37 #define NN2 0x38 #define HH2 0x39 #define OR 0x3A #define AR 0x3B #define YR 0x3C #define GG2 0x3D #define EL 0x3E #define BB2 0x3F |
Hi there
I am also playing with an old SP0256
I would love to see your code
can you post it ?
many thanks
Sure thing. I’ll try to get it posted a bit later today.
Hello,
I am currently working on a project to get a robot to ‘talk’. I have the SPO chip and was hoping for your help. I saw some of your code and was wondering if their was any chance to see all of the code. While I am good at circuit design, I am terrible when it comes to programming. I would be be extremely grateful.
Thank You,
David
FYI: I’ve updated the post to include the required loop() method (which I’d accidentally left out) and to rename the “SS” constant so it doesn’t conflict with the Slave Select constant in case that’s defined.
I would possibly be interested in the board. How much?
Hi Bruce — I’m tweaking the design a bit, and then I’ll place the order. I’ll let you know when they come in — it usually takes several weeks. I’ve been selling other PCBs for around $5 or so, so that’s probably what I’ll do here as well. -Alex
Hello sir ,
I’am Seby , i have 11 years old. The code no compiling. Please help , is my project at school . Thanks sir from Seby
byte purple[] = {PP, ER1, PP, LL }; // PP was not declared… , etc
Wow, this is quite a project for an 11 year old!
Try copying the long list of allophones from the second listing and pasting them at the top of the first code file. Let me know if you still have trouble after trying this!
Yes, I have 11 years and am a member of the circle of robotics at Children’s Palace. 2 years ago I discovered robotics and I like. To end the school year I promised to lead a project – Speech Synthesis. MikroPascal work in 2 years and 6 months in Arduino. I have many diplomas and medals in robotics competitions, MiniSumo, line follower. Platform now compiles correctly, no errors but the speaker did not hear anything. Scheme used is the website …http://www.bot-thoughts.com/2010/02/sp0256-al2-speech-with-arduino.html
I do not know if is correct, the code of that page does not work. I do not know why you used your scheme?? Thank you for your kindness and please help me to start this project very important to me. My email address is … alphateamrobotics@gmail.com. With great respect, Seby
Hello sir ,
Have you read my previous message?
With great respect, Seby
Hello sir ,
Having seen various applications, I stopped the scheme in Fig. 4 . Conclusion: working but not working properly . I changed: PIN 25 directly to PIN 2 , PIN 22 to GND .
R1 and R2 = 33K , C1 and C2 = 100nf . We removed R1 and working but not working properly.
I attached 3 mp3 files , to analyze and modified your sketch.
When I press the reset button on the Arduino is heard from the speaker unintelligible sounds different to me.
If order return address is the same thing. We need your email address to send images.
With great respect , Seby
Hi
Did you ever manage to get some boards produced for the Arduino sp0256 ?
I have had a couple of the sp0256 chips for many years and have never got round to using them. My robot project based on the Dagu Rover 5 chassis, is just the place to use one.
Thanks
Peter
Hi
Looking at your code, it looks like you are using portc for communications with the sp0256. I will be communicating between seperate processors on the robot via i2c. As a result, I cannot use portc.
I will have to revert back to plan A – design and produce my own board. Although this will only be single sided.
Thanks anyway and keep up the good work.
Peter
I’d love to see a video or some photos when you’re finished!
No, I didn’t — I lost steam on that project when I tried porting it over to KiCad. I should really pick it up again, though — it’s a fun little chip.
I have dug out a Maplin Magazine from 1990, which had an article on a kit they produced at the time.
They used the standard circuit as supplied with the sp0256 datasheet, to produce their pcb layout, but these are no longer available.
I have based my pcb layout on theirs, with the addition of an on board ATmega328 and pinouts for the I2C bus connector.
I do not get much time to work on this at the moment, but when I have a finished board and software I will let you have the info.
hey, Peter I would really appreciate the schematic hat you have developed with atmega328 and associated code please 🙂
admin can you provide schematic please I have implemented many of them but unfortunately no result 🙁
Yes, good idea. I’ll try to get it posted this weekend.
Hello 🙂
I am trying to get my 256 hooked uo with the Arduino, but it doesn’t work 🙁
Any chance you can share your schematics?
Thank you in advance
saw0
Hi, I saw your project and from years I’ve been looking for an sp0256 al2 But I could not find it nowhere…Where did u found yours and can u suggest me a dealer for such a great device? Thans for your answer.
I found mine on ebay — they show up there sometimes.
Can you please provide the PCB file (or a picture in a higher resolution). Thanks.
I’d also very much like to get my hands on PCB file if at all possible. Sitting on 4 or so of these chips
Do you have any of these PCB’s left ?
No, I’m sorry — I don’t.
Could you maybe share the gerber files with us?
I noticed the pcb omits the lp filter and audio amp. The filter cuts out most of the high frequency PWM noise and makes the speech more intelligible. Given the scarcity of these ics, driving a speaker directly is risky.
Hi,
Can you email me the schematic for the board? I want to make a slightly different board sans the ATMEGA chip. Just the synth board that any MCU can send data to.
IF you did the design in EagleCAD or KICAD sending the .sch and .brd files can help me speed up the process.
After a very long time, I have revisited my own web site and subsequently this post.
I did move forward with a design, but ended up abandoning further development due to conflicting projects. This is a link to the web page on my site, that explains things.
https://scamp.citius-software.co.uk/controllers-speech.php
If anybody wants the Fritzing file for the PCB, reply to this and I will attempt to find it.